How Do You List Reverse in Python?
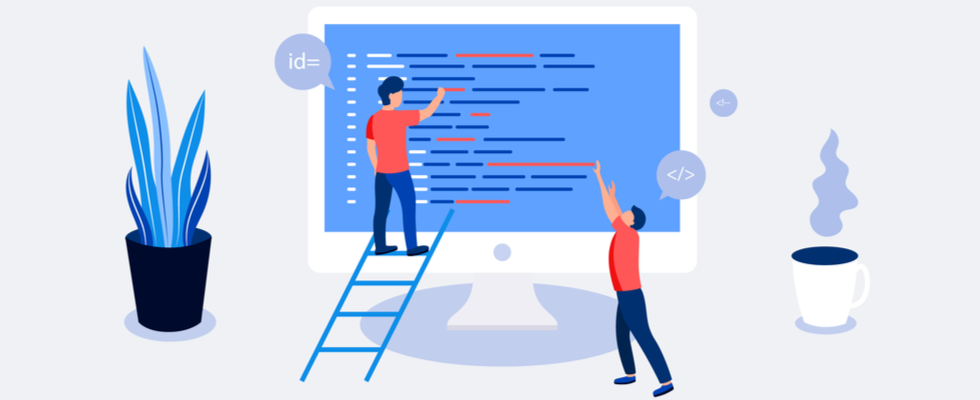
This article contains everything you need to know for how to reverse a list in Python.
Have you ever wondered how to write a program in Python that checks if a word reads the same backward as forward? Or have you simply wondered how to write your name backward with the help of Python? If your answer is yes to either of these questions, then keep reading. Here, you will learn how to use lists in Python and how to sort or reverse list elements in the desired order.
Lists are powerful data structures used in Python and many other programming languages. Once you have your elements stored in a list, you will often need to add new elements or change or delete existing elements. Lists are changeable objects, and there are several methods for altering lists in Python.
There are also methods for slicing, filtering, and finding elements. However, in this article, I’m not going to talk about these methods.
Here, you will learn about list ordering and how to use two special Python methods for element sorting and list reverse. I will explain how to use these Python functions:
- Sort()
- Sorted()
- Reverse()
Keep in mind that if you want to learn the basics of Python programming and lists, you can take Vertabelo Academy Python courses:
- Python basics — three courses that cover Python basics
- Python data structures — a course that goes deeper into lists, tuples, and dictionaries
These courses are an excellent resource for beginners.
Once you have learned the basics, let’s learn how to sort and reverse elements in a list.
Using the List.Sort() Function for List Sorting
You will often need to sort list elements – numbers from smallest to largest, characters from “a” to “z,” strings from “auto” to “zebra,” etc. Python has a built-in function, sort()
, for that purpose. There is no need to write a sort function on your own.
It is important to mention that sort()
doesn’t return an object. Instead, sort()
modifies a list. So, once you call sort()
on your list, it will modify the list by sorting the elements in ascending or descending order. Now let’s see an example of how we can use the list.sort()
.
Below are two lists of 10 elements created in Python.
The first lists 10 European countries:
countries = [‘Denmark’, ‘Croatia’, ‘United Kingdom’, ‘Iceland’, ‘Finland’, ‘Spain’, ‘Norway’, ‘Austria’, ‘Germany’, ‘Belgium’]
The second lists the average monthly gross wage in EUR for the countries listed above:
monthly_gross = [5.626, 1.139, 3.358, 5.645, 3.465, 1.971, 4.753, 4.261, 3.880, 3.558]
If you want to sort the monthly_gross
list in ascending order, you can write this:
monthly_gross.sort()
When you run this code, Python will modify the monthly_gross
list to look like this:
[1.139, 1.971, 3.358, 3.465, 3.558, 3.88, 4.261, 4.753, 5.626, 5.645]
The values are now sorted. We can now clearly see that among the 10 countries, 1139 is the smallest monthly gross wage, and 5645 is the largest one.
If you want to see the elements in descending order, you can use the sort method with the list reverse parameter.
A default value of “False” is set up for the list reverse parameter, which means that by default, elements will be sorted in ascending order.
However, if we set the list reverse parameter to “True,” the elements will be sorted in descending order. Here is how the list reverse parameter can be used on our example:
monthly_gross = [5.626, 1.139, 3.358, 5.645, 3.465, 1.971, 4.753, 4.261, 3.880, 3.558] monthly_gross.sort(reverse=True)
Python returns the elements sorted in descending order:
[5.645, 5.626, 4.753, 4.261, 3.88, 3.558, 3.465, 3.358, 1.971, 1.139]
Neat, right? So far I have shown you how to sort a list of numbers, but the list.sort()
function works with strings as well. For example, if you want to sort the countries from our example by name, then you can do this:
countries.sort()
You will get a list of the countries ordered in descending order:
[‘Austria’, ‘Belgium’, ‘Croatia’, ‘Denmark’, ‘Finland’, ‘Germany’, ‘Iceland’, ‘Norway’, ‘Spain’, ‘United Kingdom’]
There is another parameter you can specify when using the sort()
function. It is called a “key.” With a key, you can tell Python the method for sorting. For example, if you want to order string elements by their length (not by alphabetical order), you can set up the key parameter like this:
countries.sort(key=len)
In this case, the elements are not sorted in alphabetical order. Instead, they are ordered by their length (the first element has the shortest length). Keep in mind that len
is a function in Python that returns string length. The function is used on each element of the list, and the elements are ordered based on the results of the function.
Here is what happens with the countries when we use key=len
:
[‘Spain’, ‘Norway’, ‘Austria’, ‘Belgium’, ‘Croatia’, ‘Denmark’, ‘Finland’, ‘Germany’, ‘Iceland’, ‘United Kingdom’]
The countries are sorted by string length. “Spain” is the shortest string and first in the list, and “United Kingdom” is the longest string and last in the list.
Using the Sorted() Function for List Sorting
In addition to the list.sort()
function, Python has a similar built-in function, sorted()
. The main difference between the two functions is that sort()
modifies a list while sorted()
does not. Sorted()
returns a modified list, but it doesn't change the original list.
Let’s see how sorted()
works in our example. Here is our list, monthly_gross
:
monthly_gross = [5.626, 1.139, 3.358, 5.645, 3.465, 1.971, 4.753, 4.261, 3.880, 3.558]
If you want to use sorted()
, you will write something like this:
sorted(monthly_gross)
Python will display an ordered list and the original list, monthly_gross
, which has not been modified:
In [2]: sorted(monthly_gross) Out[2]: [1.139, 1.971, 3.358, 3.465, 3.558, 3.88, 4.261, 4.753, 5.626, 5.645]
Like with list.sort()
, sorted()
can also be called with the key and reverse parameters. For example, if you want to see monthly_gross
in descending order, you can call sorted()
with the reverse parameter like this:
sorted(monthly_gross, reverse = True)
This will display the list in descending order.
Sort() vs. Sorted()
The main difference between sort()
and sorted()
is that sort()
modifies the list while sorted()
does not. We saw that when calling monthly_gross.sort()
, Python modified the original list, and nothing was returned. However, when calling sorted(monthly_gross)
, Python returned an ordered list and did not modify the original list, monthly_gross
.
Using the List.Reverse() Function to List Reverse
Another important and interesting function that changes the order of elements in a list is reverse()
. This function reverses the order of elements in a list—the first element is put in the last spot, the second element is put in the second to last spot, and so on (we call this “list reverse”).
It is easy to use the reverse()
method because a list reverse is always called without any parameter. Also, it doesn’t return anything?—?it just modifies the existing list to reverse the elements. So, if we use the reverse method on our countries list by writing this:
countries.reverse()
This is what happens:
As you can see from the image, Denmark moves to the last place and Belgium to the first. We reversed the order of the elements, a list reverse. This example shows you how to reverse a list of strings, but of course, the same thing can be done with a list of numbers. By typing this:
monthly_gross.reverse()
Our gross salaries list is reversed:
The list has been modified so that the numbers now are in reverse order.
Although using the reverse()
function is relatively easy, instead of using this built-in function, you can also use other methods. In the next section, we will talk about alternatives—for
and while
loops and a slicing operator.
Using a While or For Loop to List Reverse
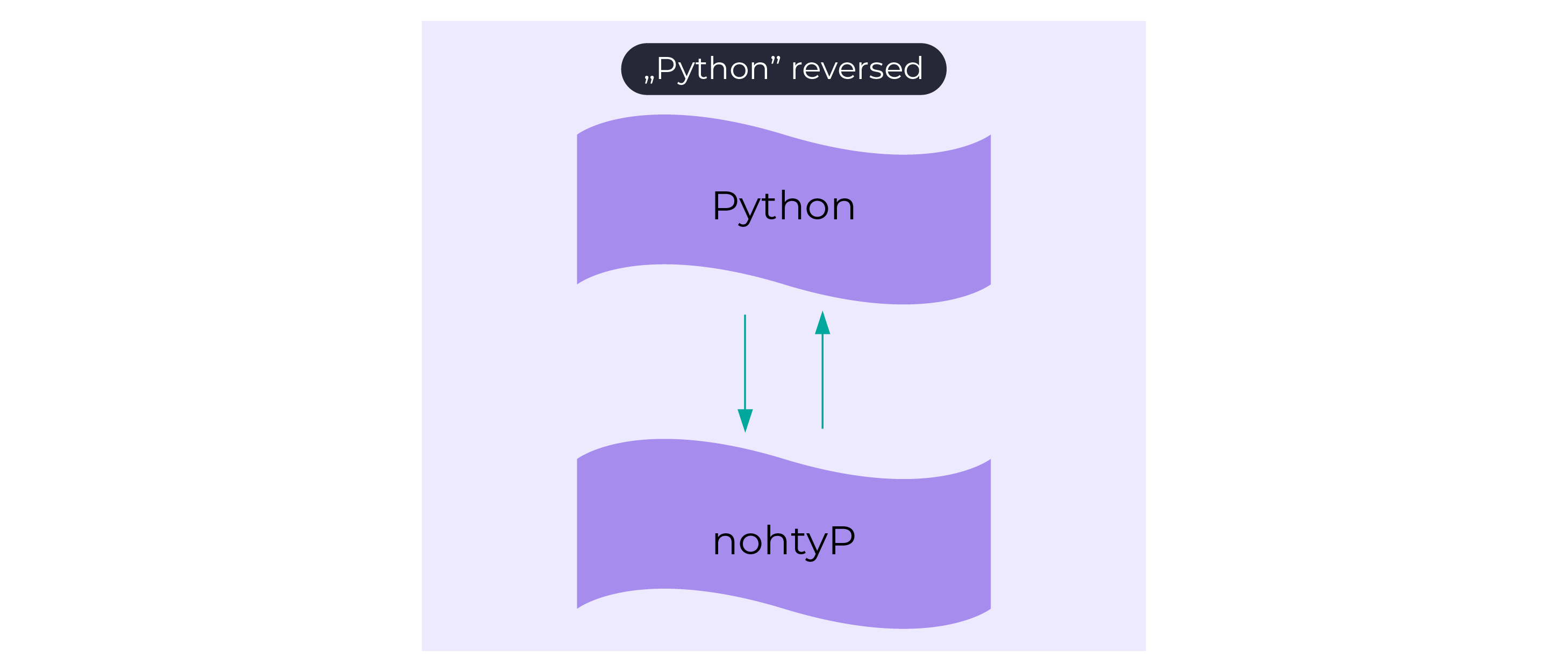
Have you ever wondered how to reverse a list in Python without using reverse()
? If you want to avoid direct modification of an original list, you can reverse a list by using a while loop. We already know that each element in a list can be accessed by using an index. For example, the first element of the list countries
can be accessed with countries[0]
, the second one with countries[1]
, and the last one with countries[9]
. So, if you want to print a list in reverse order, start from the last element and iterate through the whole list until the first element in a list is not printed or displayed.
Here is the while
loop for printing the countries in reverse order:
countries = ['Denmark', 'Croatia', 'United Kingdom', 'Iceland', 'Finland', 'Spain', 'Norway', 'Austria', 'Germany', 'Belgium'] position = len(countries) — 1 while(position>=0): print(countries[position]) position = position -1
First, we created a position variable with the help of the len function. Before entering the while loop, the value of the position variable is 9, the last element in the list. I mentioned earlier that the list of countries contains 10 elements, but because the indexes are starting from 0, the last element is countries[9]
, not with countries[10]
. Next, you need to iterate through the list from 9 to 0 and print each element (notice how in each iteration, the position is decreased by one). This is how you can print the elements in reverse order without using the reverse()
method.
Something similar can be done with a for loop:
countries = ['Denmark', 'Croatia', 'United Kingdom', 'Iceland', 'Finland', 'Spain', 'Norway', 'Austria', 'Germany', 'Belgium'] for i in [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]: print(countries[i])
This reverses the list by using a for loop. In the for
loop, we defined a range of indexes?—?starting again from the last index and stopping at 0. Here, we explicitly said that i
has the values 9, 8, 7, .., 0, but we can specify the same numbers by using the range
function (and avoid difficult coding):
for i in range(len(countries)-1, -1, -1): print(countries[i])
The result is the same as with the for
loop above except that we have defined a range for the iterator i by using the functions range
and len
(we did not use hard-coded values as in the previous for
loop).
Using the Index Function to List Reverse
Besides using while
and for
loops or the reverse()
function, it is also possible to use the indexing operator for slicing lists. The syntax for slicing list elements using indexes is this: list[start:stop:step].
- “Start” denotes the index of the first element that you want to retrieve
- “Stop” denotes the index of the last element that you want to retrieve
- “Step” denotes the increment between each index for slicing
For example, if we type countries[1:5:2]
, Python will print Croatia and Iceland. Here is why:
You can see that by calling countries[1:5:2]
, Python looks at the elements at indexes 1, 2, 3, and 4 and prints out the elements at positions 1 and 3 (Croatia and Iceland). Position 5 is the index at which Python stops. Therefore, the country at position 5 is not taken into consideration.
A similar syntax could be used with negative indexes. For example, by calling countries[5:1:-1]
, Python will return a sub-list of the elements at positions 5, 4, 3, and 2. The step is -1, so the starting index is 5 (Spain), and the stopping index is 1 (Croatia), which is not included in the sub-list:
If you want to list reverse a whole list, there is no need to define starting and stopping positions. By calling countries[::-1]
, Python knows that the starting point is the last element in the list and the stopping point is the first element. Because the step is -1, Python reads the elements from the last element until the first element in the list.
This output is the same as with the reverse()
method, except that the original list is not modified.
Summary
There are many built-in functions for working with lists in Python. If you are not familiar with Python lists and basic data structures, I suggest trying out Vertabelo’s interactive courses for beginners: Python Basics Part1, Part2, and Part3. They are great for people interested in data science and data analytics with no prior programming experience in Python.
In this article, you learned which functions to use when you need to change the order of the elements in a list (like a list reverse or element sorting in ascending/descending order).
Two common functions for element sorting are sort()
and sorted()
. With both functions, you can sort list elements in ascending or descending order. But there is one major difference: sort()
modifies the original list while sorted()
does not. Also, both functions can be called with an additional parameter, reverse
, which can be set to “True” or “False” for descending or ascending order.
Another function that changes the order of elements in a list is reverse()
. It is used when you want to reverse elements in a list (a list reverse).
You also learned how to list reverse without using built-in functions. Using while
and for
loops and the indexing operator are viable options.
After learning all of these methods for reversing the order of elements in a list, are you convinced that using reverse()
is the most elegant and simple?