The Vertabelo Guide to Python Operators
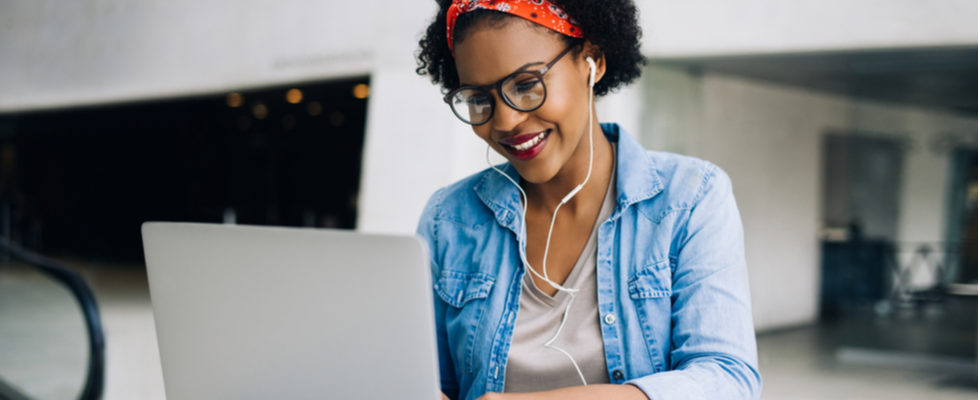
Want to become proficient with Python operators? In this comprehensive guide, you’ll learn everything you need to know about the types of operators in Python, their differences, and syntax and explore cases with easy-to-follow code examples.
What Are Operators in Python?
Operators are special symbols used to perform different operations on variables and values. The variable or value that the operator operates on is called the operand.
In Python, operators are divided into the following groups:
- Arithmetic operators
- Comparison (relational) operators
- Logical (Boolean) operators
- Bitwise operators
- Assignment operators
- Identity operators
- Membership operators
In this guide, we are going to go through all of these types of Python operators with explanations of the operators from these groups, syntax nuances, and code examples.
Let’s start!
Python Arithmetic Operators
Arithmetic operators in Python are used with numerical values to perform mathematical operations.
OPERATOR | EXPLANATION | SYNTAX |
---|---|---|
+ | Returns sum of the operands | a + b |
- | Returns difference of the operands | a - b |
* | Returns product of the operands | a * b |
/ | Divides the first operand by the second one | a / b |
// | Divides the first operand by the second with the result rounded to the lower whole number | a // b |
% | Returns the remainder from the division of the first operand by the second one | a % b |
** | Raises the first operand to the power of the second one | a ** b |
While operations like addition, subtraction, multiplication, and division probably do not need additional explanation, it would be nice to see how other arithmetic operators behave in practice.
# Arithmetic operators print(25//2) print(25%2) print(25**2)
12 1 625
As you can see from the above examples, the floor division (//
) of 25 by 2 returns 12, the modulus (%
) from the same values returns 1, which is the remainder of this division, and the exponentiation (**
) simply takes 25 to the power of 2, resulting in 625.
Python Comparison Operators
Comparison operators, also known as relational operators, are used to compare the values of operands.
OPERATOR | EXPLANATION | SYNTAX |
---|---|---|
> | Returns “True” if the value of the first operand is greater than the value of the second one | a > b |
< | Returns “True” if the value of the second operand is greater than the value of the first one | a < b |
>= | Returns “True” if the value of the first operand is greater than or equal to the value of the second one | a >= b |
<= | Returns “True” if the value of the second operand is greater than or equal to the value of the first one | a <= b |
== | Returns “True” if the values of both operands are equal | a == b |
!= | Returns “True” if the values of the operands are not equal | a != b |
To see how these comparison operators look in Python code, let’s compare the number of Oscar awards received by four prominent actors and actresses: Katharine Hepburn – 4 awards, Meryl Streep – 3 awards, Jack Nicholson – 3 awards, and Robert De Niro – 2 awards.
# Comparison operators Hepburn = 4 Streep = 3 Nicholson = 3 De_Niro = 2 print (Hepburn > Streep) print (Nicholson < Streep) print (Nicholson >= Streep) print (De_Niro <= Nicholson) print (Streep == Nicholson) print (Nicholson != De_Niro)
True False True True True True
Now you can check your understanding by seeing if all operators behave as you would expect.
Python Logical Operators
Logical operators, also known as Boolean operators, are used to combine conditional statements and create even more complex conditions.
OPERATOR | EXPLANATION | SYNTAX |
---|---|---|
and | Returns “True” if both operands are true | a and b |
or | Returns “True” if at least one of the operands is true | a or b |
not | Returns “True” if the operand is false, and vice versa | not a |
# Logical operators (Boolean Operands) print (Hepburn > Streep and Nicholson < Streep) print (Hepburn > Streep or Nicholson < Streep) print (not Hepburn > Streep)
False True False
Interestingly, the operands do not need to be of the Boolean type to be used with the Boolean operators. You can use these operators with numerical values, strings, and composite data types like lists, sets, tuples, and dictionaries. For each of these data types, you should be able to say if the corresponding value is “True” or “False.”
Doesn’t sound like an easy task? Don’t worry—in Python, evaluation of non-Boolean values in Boolean context is well-defined. The following values are always considered false in the Boolean context:
- Numerical values that are equal to zero
- Empty strings
- Empty lists, sets, tuples, and dictionaries
- “None” values
All the other values will be considered true in the Boolean context. The built-in bool()
function allows you to determine if a certain value is considered “True” or “False.”
Ready for the code examples?
# Logical operators (non-Boolean values) print (bool(25), bool("age"), bool(25 and "age")) print (bool(0), bool(None), bool(0 or None)) print (bool([]), bool([1,2,3]), bool(not []))
True True True False False False False True True
Python Bitwise Operators
Bitwise operators manipulate operands as if they were strings of binary digits. These operators perform operations bit by bit. It’s easier to understand with examples.
Let’s take a = 12 or 0000 1100 in binary, and b = 7 or 0000 0111 in binary. Now we are ready to explore the output of the bitwise operators through examples.
OPERATOR | EXPLANATION | SYNTAX |
---|---|---|
& | Bitwise AND: copies a bit to the resulting value if it exists in both operands | a & b = 4 (0000 0100) |
| | Bitwise OR: copies a bit to the resulting value if it exists in at least one of the operands | a | b = 15 (0000 1111) |
^ | Bitwise XOR: copies a bit to the resulting value if it exists in one operand but not in another | a ^ b = 11 (0000 1011) |
~ | Bitwise NOT: flips the bits in the operand | ~ a = -13 (1111 0011) |
>> | Bitwise right shift: the value of the left-side operand is moved right by the number of bits specified by the right-side operand | a >> 1 = 6 (0000 0110) |
<< | Bitwise left shift: the value of the left-side operand is moved left by the number of bits specified by the right-side operand | a << 1 = 24 (0001 1000) |
Performing operations bit by bit manually is quite a meticulous task prone to mistakes. Let’s use Python to check if our calculations in the table above are correct.
# Bitwise operators a = 12 b = 7 print (a & b) print (a | b) print (a ^ b) print (~ a) print (a >> 1) print (a << 1)
4 15 11 -13 6 24
Huh, it looks like everything is correct!
Python Assignment Operators
Assignment operators in Python are used to assign values to variables.
OPERATOR | EXPLANATION | SYNTAX |
---|---|---|
= | Assigns the value of the right side of expression to the left-side operand | a = b + 5 |
+= | Assigns the sum of the left-side operand and the right-side operand to the left-side operand | a += b (a = a + b) |
-= | Subtracts the right-side operand from the left-side operand and assigns this value to the left-side operand | a -= b (a = a - b) |
*= | Assigns the product of the left-side operand and the right-side operand to the left-side operand | a *= b (a = a * b) |
/= | Divides the left-side operand by the right-side operand and assigns this value to the left-side operand | a /= b (a = a / b) |
%= | Assigns the remainder from the division of the left-side operand by the right-side one to the left-side operand | a %= b (a = a % b) |
//= | Divides the left operand by the right operand with the result rounded to the lower whole number and assigns this value to the left-side operand | a //= b (a = a // b) |
**= | Raises the left-side operand to the power of the right-side operand and assigns this value to the left-side operand | a **= b (a = a ** b) |
&= | Assigns the value from the bitwise AND operation to the left-side operand | a &= b (a = a & b) |
|= | Assigns the value from the bitwise OR operation to the left-side operand | a |= b (a = a | b) |
^= | Assigns the value from the bitwise XOR operation to the left-side operand | a ^= b (a = a ^ b) |
>>= | Assigns the value from the bitwise right shift operation to the left-side operand | a >>= b (a = a >> b) |
<<= | Assigns the value from the bitwise left shift operation to the left-side operand | a <<= b (a = a << b) |
As you can see, assignment operators just take the output of the arithmetic and bitwise operators and assign the corresponding value to the left-side operand. Let’s check how they behave in Python code.
# Assignment operators a = 12 b = 7 a += b print (a) a //= b print (a) a &= b print(a) a ^= b print(a)
19 2 2 5
Python Identity Operators
There are only two identity operators in Python, and they are used to check if two variables are identical or, in other words, located in the same part of the memory. Please note that even if two variables are equal, they are not necessarily identical, according to this definition.
OPERATOR | EXPLANATION | SYNTAX |
---|---|---|
is | Returns “True” if the operands are identical (refer to the same object) | a is b |
is not | Returns “True” if the operands are not identical (do not refer to the same object) | a is not b |
Let’s see the difference between equal and identical variables with some code examples.
# Identity operators a = 12 b = 5 + 7 print (a is b) print (id(a), id(b)) list_1 = ["apples", "oranges", "cherries"] list_2 = ["apples", "oranges", "cherries"] print (list_1 is list_2) print (id(list_1), id(list_2))
True 4303754928 4303754928 False 4350704904 4350072968
As you can see from the output of our code, variables of immutable data types, such as numerical values, are considered identical when equal. The identity of the variables can also be verified using an id()
function, which indicates if these variables are located in the same part of the memory.
This doesn’t work for the mutable data types such as lists. As you can see in the example, if the same list is assigned to two different variables, two separate objects are created.
Python Membership Operators
In Python, we also have two membership operators that are used to check whether a value or variable is found in the sequence. This sequence can be of different data types, including lists, strings, tuples, sets, and dictionaries. However, note that in the case of a dictionary, we can only test for the presence of key but not value.
OPERATOR | EXPLANATION | SYNTAX |
---|---|---|
in | Returns “True” if the value of the first operand is found in the sequence (second operand) | a in b |
not in | Returns “True” if the value of the first operand is not found in the sequence (second operand) | a not in b |
Now we are ready to check how membership operators behave in Python code.
# Membership operators our_list = ["apples", "oranges", "cherries"] our_dict = {"apples":"fruits", "oranges":"fruits", "cherries":"berries"} print ("apples" in our_list) print ("apples" in our_dict) print ("fruits" in our_dict)
True True False
As expected, the value “fruits” was not found in the dictionary because the membership operator tests only for the presence of keys.
It’s Time to Practice with Python Operators!
You should now have a good grasp of operators in Python. But there are so many of them! You need to spend some time practicing to become fully proficient with all the operators available in Python.
Dozens of interactive exercises available in our Python Basics. Part 1 and Python Basics. Part 2 courses will help you strengthen your newly acquired knowledge of Python operators. Practice with arithmetic operators, comparison operators, logical operators, assignment operators, membership operators, and more.
Thanks for reading, and happy learning!