Getting Started with Python, Part 2: Python Variables
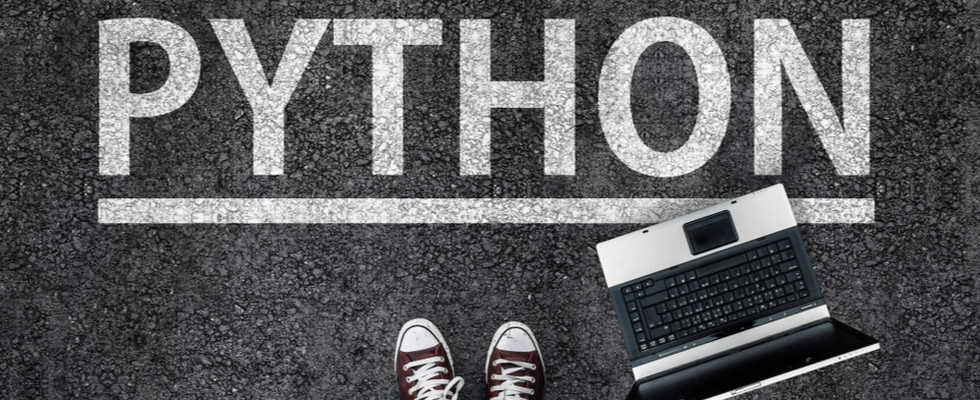
Want to learn what Python variables are and what you can do with them? That’s exactly what we’ll cover in this article.
Now that you’re at Part 2 of our Python series, it’s time to learn about Python variables. (Yes, Part 2 means there is also a Part 1. For those who missed it, Part 1 discusses Python’s data types and some basic mathematical operations. It also introduces the concept of a function.)
Now, let’s build on our knowledge and find out what variables are. We'll also learn the difference between global and local variables and how to declare them, re-declare them, and delete them.
What Is a Variable?
Simply put, Python variables are places in computer memory that have names assigned to them. Those places are used for storing data. Since data is always stored in a specific format (i.e. in a data type), you can name and store any of the data types from the previous article. The main point of variables is that you can easily retrieve the stored data any time you want.
Python Variables Types
Python has two types of variables:
- Global variables
- Local variables
Global variables are variables that are declared outside a function. They can be used by everyone, no matter if it’s inside or outside a function. (Remember, functions are reusable blocks of organized code that perform a certain action.) All Python variables are global by default unless the user indicates differently.
Local variables are declared inside a function. They can only be used inside that function.
It seems that I've also mentioned something non-familiar: declaring a variable. Let's see what that is!
Using Actions on Variables
There are main actions that you can use on variables:
- Declaring a variable.
- Re-declaring a variable.
- Deleting a variable.
Handling a Global Variable in Python
Variable declaration
Declaring a variable is the same as creating a variable. Declaring a variable consists of two parts:
- Naming the variable.
- Assigning a value to a variable.
Naming a global variable
Variable names are not length restricted. They can contain letters (uppercase or lowercase), digits (0-9), and the underscore character (_). To put it more precisely, an underscore is used as a space. A variable name must have an underscore if it has more than one word or to separate signs.
Variable names have some restrictions. A variable name must start with either a letter or an underscore; the variable name can't start with a digit. Also, a variable cannot use a reserved word (a keyword) as its name. Reserved words are used by Python to indicate a certain functionality. To see the list of the Python keywords, run the following code:
help ('keywords')
You’ll get a list of 33 keywords, as shown below:
Here is a list of the Python keywords. Enter any keyword to get more help. False def if raise None del import return True elif in try and else is while as except lambda with assert finally nonlocal yield break for not class from or continue global pass
And, almost by accident, you've learned to use another function: the help function!
Assigning a value to a global variable
After naming a variable, it's time to assign a value to it. You can use any data type supported by Python. Since you already know something about text and numeric data types, now is the time to use that knowledge.
After learning how to declare a variable in theory, let’s use it in practice.
If you don't want to install Python on your computer, you can practice variable declaration in this part of our Python Basics course.
After you're done, skip to the next section of this article, where you’ll learn how to re-declare a global variable.
To declare a variable, use the following code:
feeling = 'Currently feeling great'
By running this code, you will define a variable named feeling
. It will have the value “Currently feeling great” assigned to it. In Python, the equals sign (=
) means “assign the value of”. Simple, isn't it?
To retrieve the data, use this code:
print (feeling)
Your output will be “Currently feeling great”.
I've mentioned that variable names can contain multiple words, so let's see how that works. Use the following code to declare a new variable:
current feeling = 'Great!'
What did you get, an error? Perfect – that was my intention! Remember, a variable name can contain several words, but they have to be separated by an underscore (_
). Let's correct the error this way:
current_feeling = 'Great!'
Now that you've declared the variable, show its value by using the print function:
print (Current_feeling)
Get another error? There's a message saying “NameError: name 'Current_feeling' is not defined”. How is that variable not defined when we just defined it a few seconds ago?!
Be careful when defining variables: Python is case-sensitive. This means that current_feeling
and Current_feeling
are two different variables in Python. It’s good coding practice to use only lowercase when writing code and separate the words by an underscore. Let's correct this and show the value of the real variable, the one that we've defined:
print (current_feeling)
See, it works perfectly! Let's switch things up and declare a variable with a numeric value. The approach is the same as above. By running the following code ...
height = 175
… you’ll define a variable named height and the value assigned to it will be 175
. This is a numeric value – an integer, more specifically.
If you have several variables that you want to declare, there's no need to declare every variable separately. You can do it in one line of code, like this:
year_born, weight, zodiac_sign = 1982, 77, 'Sagittarius'
To check if you did it right, retrieve the data:
print(year_born) print(weight) print(zodiac_sign)
Of course, a shorter version can be used, too:
print(year_born, weight, zodiac_sign)
Re-declaring a global variable
After you’ve learned how to declare a global variable, to re-declare it is very easy. Re-declaring is nothing more than assigning a new value to an already-declared variable. Let’s practice this a little bit!
Psst! The section of our Python course that I mentioned before includes re-declaring variables, so feel free to practice there.
Let's say you assigned the wrong data to a variable. You want to change the value from 175 to 178. What you will do is re-declare the variable. Simply run the code ...
height = 178
… and it will change the variable value to 178. To check if you succeeded, retrieve the data by running the code:
print(height)
One of the great things about Python is that you can assign one data type to a variable and later change that variable to a different data type. In many programming languages, once a variable’s data type is defined, all assigned values have to be of that type. In Python, not only can you change a variable’s value, you can also change its data type. Let's say you don't have height data and you want your variable to show it. Run this code:
height = 'No data available'
The height value and type will be changed. To check all this, run the complete code with all its changes:
height = 175 height = 178 print(height) height = 'No data available' print(height)
It will show you three values for the same variable: The initial one and all subsequent changes.
Deleting a global variable
Deleting a variable is as simple as declaring or re-declaring it. To delete a variable, simply use the function del
.
For example, let's delete the latest variable you declared, which is the height
variable. Run this code:
del height
The variable height will be deleted. If you don't trust me, maybe you will trust Python. Try to retrieve the data ...
print(height)
… and Python will return the message “NameError: name 'height' is not defined”.
Now that you've learned how to use the del
, help
, and print
functions, it's time to learn how to declare a local variable.
Handling a Local Variable in Python
Declaring a local variable
To declare a local variable – a variable that’s only used within a function – you first have to define the function. We’ll talk more about user-built functions in the future, so don't be scared. This is just to show you how to declare a local variable and get familiar with what a user-built function looks like.
You define a function by using the def
command. By running the following code ...
def example(): height = 175 print(height) example()
… you will define a function named example
. The start of the function is marked by example()
: and the function ends with example()
. In between is the code that defines what you want the function to do. In this case, you defined the local variable height with a value of 175 and told the function to print the variable’s value.
Let's try to retrieve the value of the variable outside the function. Run the following code and see what happens. (It’s the same as above, only with a second print
function.)
def example(): height = 175 print(height) example() print(height)
You get 175, which is the function variable. But you also get the message “NameError: name 'height' is not defined”. This is because, as I mentioned, the local variable can only be used within a function. If you try to use it outside the function, there will be an error because a global variable is not defined.
Let's change the above code so that it contains both local and global variables. Above the print function, I've declared a global variable. The complete code looks like this:
def example(): height = 175 print(height) example() height = 189 print(height)
If you run the code, you will get both 175 and 189 – the values of both the local variable and the global variable. Now you understand the difference between those two variables and know how to combine them.
Re-declaring local variables
Of course, you can re-declare a local variable the same way you re-declare a global one. If you want to re-declare your local height variable and change its value to 178, you do it like this:
def example(): height = 175 print(height) height = 178 print(height) example()
It's the same as with a global variable, except this is done within a function.
Python assumes that every variable declared within a function is by default a local variable. Nevertheless, you can change the local variable within the function. And you can change global variables, too! But you have to specifically state that by using the keyword global, which means you're referencing a global value inside the function. (Remember, there are 33 keywords that variables can't be named after. “Global” is one of those reserved words.)
Let's see how it works! Run this code:
height = 180 print(height) def example(): height = 195 print(height) global height height = 'This is changed global variable' example() print(height)
You will get the following result: 180, 195. This is the changed global variable. Let's analyze the code!
First, I've declared a global variable height and assigned it the value 180. Then I've created a function named example with the local variable height with the value 195. Next, I've changed the value of the global variable by writing global next to the height variable. This means I'm referencing the global variable, not the local variable of the same name. Then I re-declared this global variable. In the end, I've retrieved the global variable and returned the value. This value is changed according to the command written in the code.
Let's now see if you can delete a local variable the same way as you deleted a global variable.
Deleting local variable
You used del function to delete the global variable. Let’s see if the same function will delete the local variable.
I've changed the code above and added a line of code that deletes the height variable and then tries to retrieve its value. The code looks like this:
def example(): height = 175 print(height) height = 178 print(height) del height print(height) example()
Run the code and see what you get. If you've done everything right, you should get 175, then 178, and then the message “UnboundLocalError: local variable 'height' referenced before assignment”. This means you've successfully deleted the local variable.
Now That You Know Python Variables
In this part, you’ve learned what Python variables are. You’ve learned the difference between global and local variables. Also, you know how to declare, re-declare, and delete variables. In the process of learning that, you’ve also learned three new functions: help
, def
, and del
. Besides those built-in functions, you've learned how to create a function.
As in the previous article, I've used the Vertabelo Python course to help me understand all this. Give it a try if you want to learn more!
How do you like this Python article series? Do you find it helpful in learning Python basics? Feel free to let us know in the comments section. We’d like to hear from you so that we can adapt the next article(s) to your needs. The main point is to help you learn most efficiently. Feel free to have your say!