Lists and List Comprehension in Python
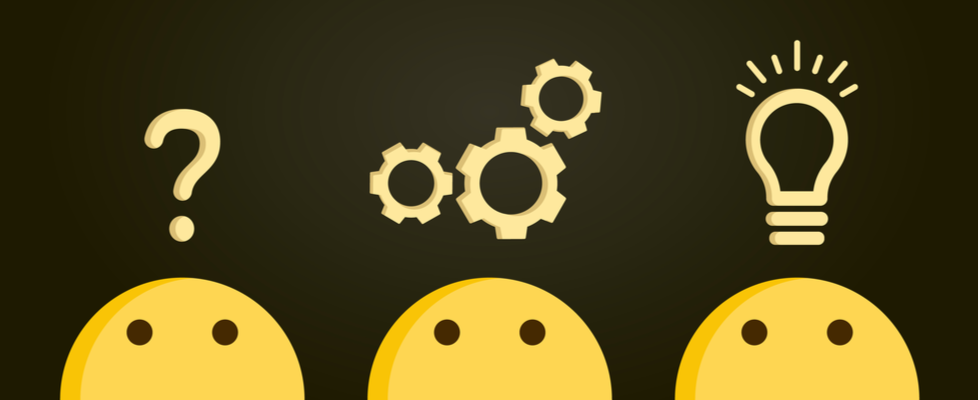
Everything you need to get started with lists and list comprehension in Python.
Here comes the New Year — the perfect time for your own personal re-evaluation and a retrospective of the last year. It’s also a great time to sit down and write a list of New Year's resolutions that you are planning to achieve in the incoming year. Are you going to write it on paper or will you reach for your laptop and, like any other nerd, use Excel or some other tool like Python to save and maintain this list?
We’ll assume that you like Python. In this article, you’ll learn how to create and modify a list in Python. You will also learn how to use the list comprehension construct to create a list from a list that already exists.
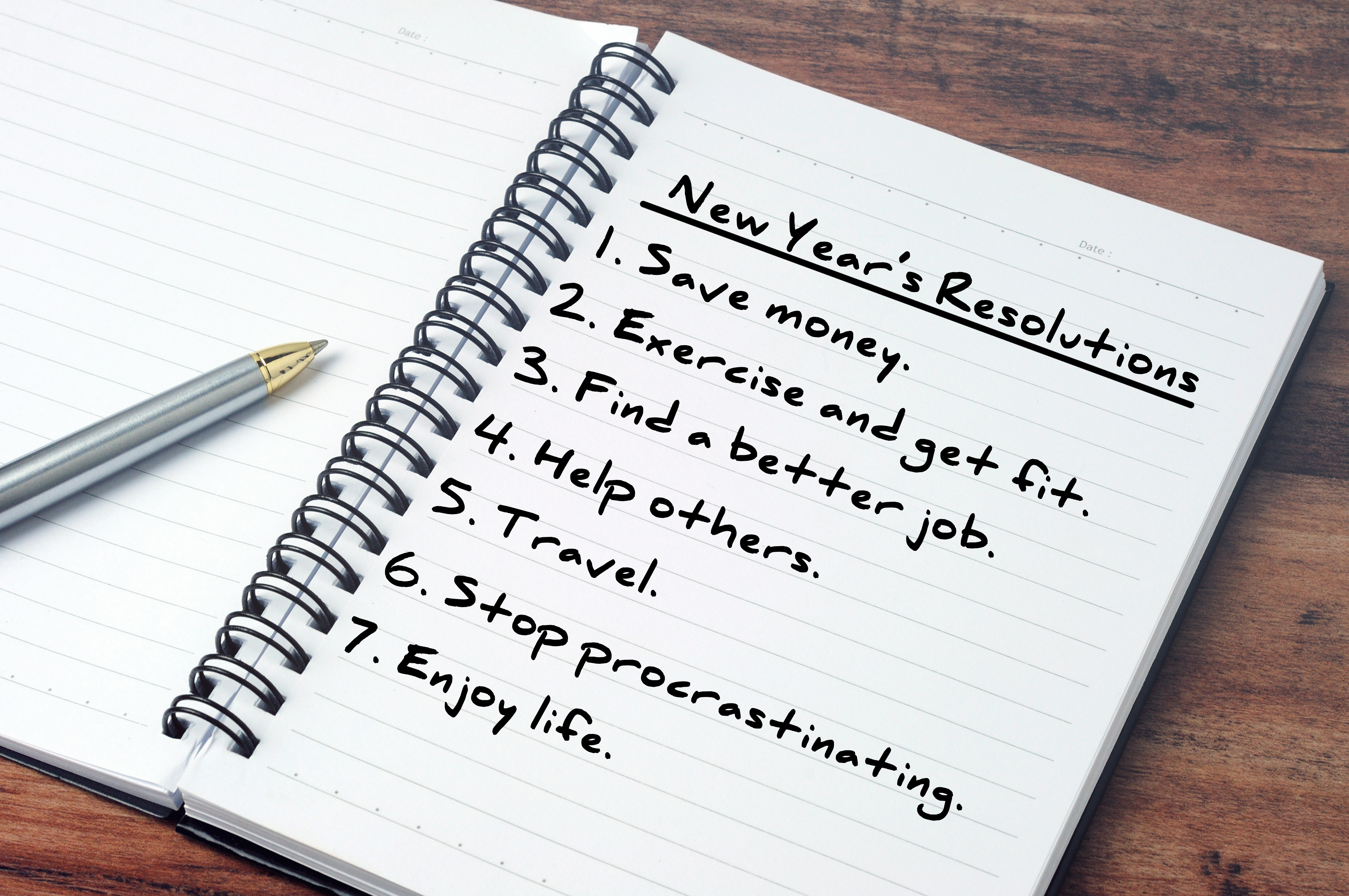
Lists and List Creation in Python: The Basics
One of the most famous and most used collection objects or sequences in Python are lists. In Python, a list can be a collection of strings (like a New Year’s resolution list) or a collection of numbers (like a list of your weight for each month of the last year). Lists can also be more complex, such as a collection of other objects. You could even make a list of other lists.
Here is one example of a list of strings. Note how it is created in Python:
NewYearResolution = [“stop smoking”, “lose weight”, “join gym”, “find a new job”,”enjoy life”]
In our example, “NewYearResolution” is the list name. Each element in this list is a string. Inside square brackets [ ], list elements are defined and separated with a comma. Our list has 5 resolutions (i.e. it is a list of 5 elements). Each resolution (element) is indexed with a number (0,1,2,3 or 4). The first element, “stop smoking”, has an index of 0 and can be reached with the command NewYearResolution[0]. The second element, “lose weight”, has an index of 1 and can be reached with NewYearResolution[1], and so on. The last element, “enjoy life”, can be reached with NewYearResolution[4].
This is how to create a list in Python and access each list element. If you are not familiar with lists and other Python objects and you want to do some more studying, check out Vertabelo Academy’s interactive online courses for Python beginners:
The Vertabelo Python Data Structures course explains Python’s data structures (including lists); this could be useful for you as a next step after acquiring basic Python skills.
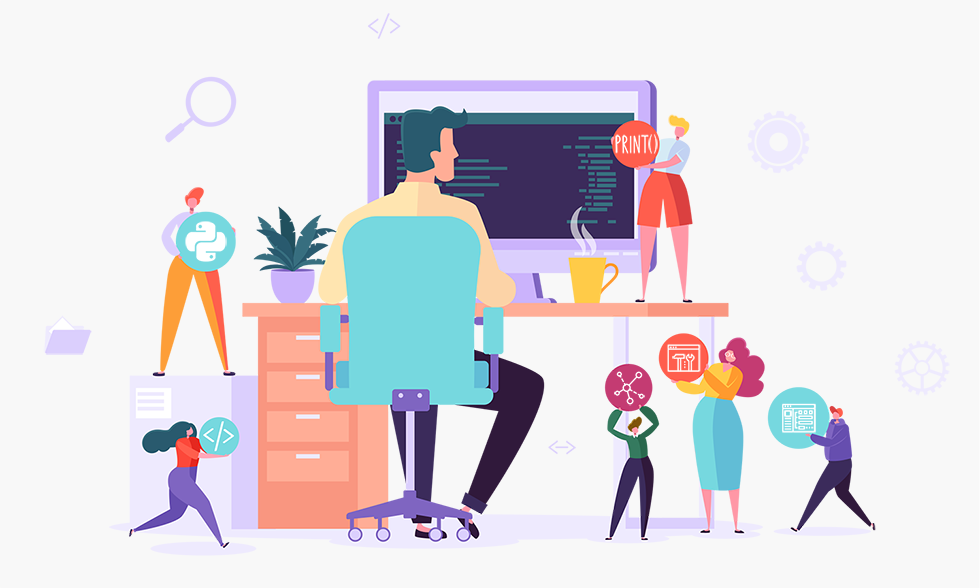
Working with Python Lists
Lists are changeable objects
It’s important to know that lists are changeable — you can add, remove, or change list elements after the list is created.
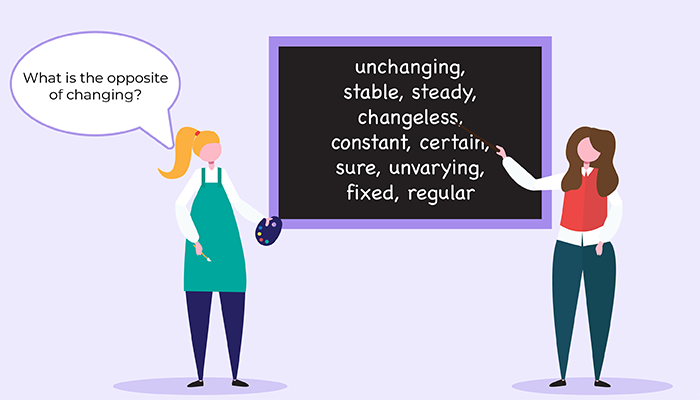
Lists are changeable objects
For example, if you want to change the fifth element in the list NewYearResolution to “reduce stress”, you can simply do this:
NewYearResolution[4]=”reduce stress”
We are telling Python that the fifth element (the one with index 4) should be defined as “reduce stress”. After this statement is run, our list is modified and now looks like this:
List after modifying the fifth element
Inserting and removing elements from an existing list
Of course, we mentioned you can also add or remove elements from a list.
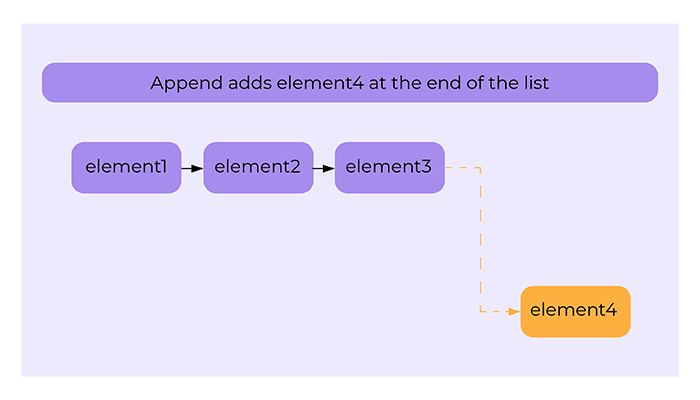
The append() function adds an element to the end of a list
If you want to add another resolution to the NewYearResolution list, you can do that by calling the append() function:
NewYearResolution.append(“get more sleep”)
Now, if you type NewYearResolution, Python returns the following in the output screen:
['stop smoking', 'lose weight', 'join gym', 'find a new job', 'reduce stress',’get more sleep’]
Adding an element to the last position
As you may have noticed, the new resolution is in the last position on the list. The append() function always appends an element, or adds it at the end. If you don't want the new element in the last position, you can use the insert() function to specify exactly where you want to place your element.
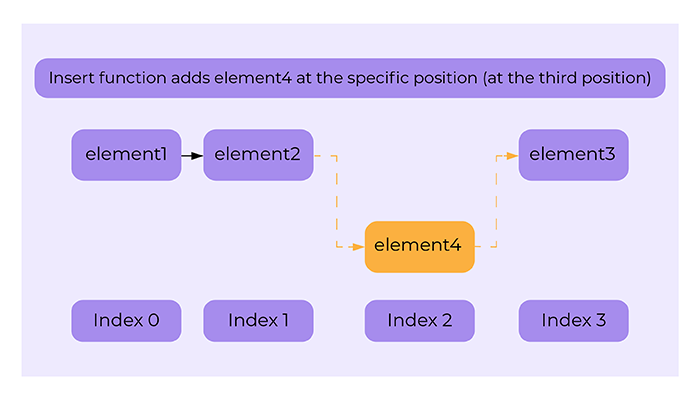
The insert() function adds an element in a specific position
For example, if you want to put the resolution “eat healthy” in the third place, you’d call insert() like this:
NewYearResolution.insert(2,”eat healthy”)
The first argument is 2, which is the index position where you want to place the “eat healthy” element. This means that “eat healthy” will be presented as the third element in the list.
Beside inserting an element, you can also remove elements. Simply use the remove() function, like this:
NewYearResolution.remove(“join gym”)
After you call this statement, Python will permanently remove “join gym” from the list.
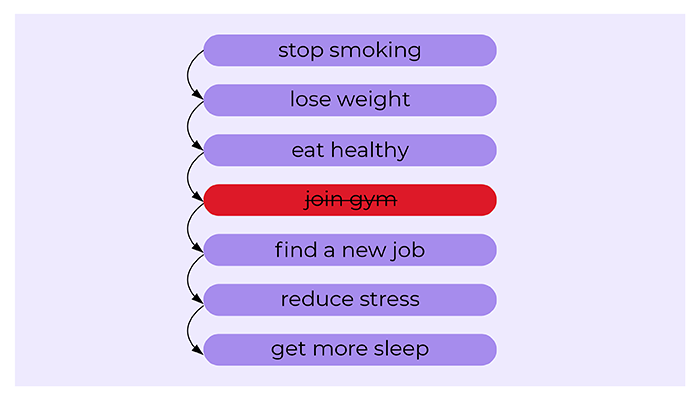
Removing “join gym” from the list
Iterating through a list
Adding, removing, or changing one element is easy, but what happens when you want to apply some changes or transformations to every list element? In that case, you need to iterate through a whole sequence; you can do this using a for loop.
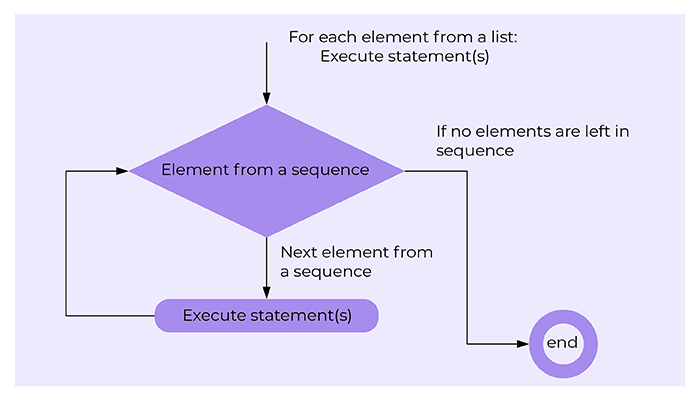
Flow diagram of a for loop
Here is one example how you can print each element from the NewYearResolution list with a for loop:
for resolution in NewYearResolution: print(resolution)
We are iterating through the whole list and printing the content of each element to the output console. We’re using an existing list and applying a print() command to each element. In this case, we didn’t create a list or change any list elements — we just went through a whole list and printed out the content.
We can also use a for loop to create a list based on an existing list(s). Let's go through a simple example using a list of the highest paid actors of 2019. Here’s the list:
highest_paid_actors = [“Will Smith”, “Paul Rudd” ,”Chris Evans” ,”Adam Sandler” ,”Bradley Cooper”,”Jackie Chan” ,”Akshay Kumar” ,”Robert Downey Jr.” ,”Chris Hemsworth” ,”Dwayne Johnson” ]
Now let's take a look at their respective birth dates. (Some of them are not even 40 and have already earned so much!)
highest_paid_actors_birthdate = [“25.9.1968”,”6.4.1969",”13.6.1981",”9.9.1966",”5.1.1975",”7.4.1954",”9.9.1967",”4.4.1965",”11.8.1983",”2.5.1972"]
Let's assume that we want to extract the year from each actor’s birthdate and save that in another list named highest_paid_actors_birthyear. Here is a for loop that does it:
import datetime highest_paid_actors_birthyear=[] for birthdate in highest_paid_actors_birthdate: birthdate_year = datetime.datetime.strptime(birthdate,"%d.%m.%Y").year highest_paid_actors_birthyear.append(birthdate_year)
We first initialized an empty list named highest_paid_actors_birthyear:
We first initialized an empty list named highest_paid_actors_birthyear:
Then we used a for loop to iterate through the entire sequence. For each birthdate, we ran a statement that converts the birthdate string into a datetime (a data type that includes date and time info) and extracts the year:
datetime.datetime.strptime(birthdate,”%d.%m.%Y”).year
For this extraction, we used the functions strptime() and year, which are part of Python’s datetime module. Because we’re working with dates and need these functions, we first import the datetime module to access them. Then we can use the functions.
The year value is then stored in the birthdate_year variable, which is then appended to a new list named highest_paid_actors_birthyear. After this for loop is run, our newly created list highest_paid_actors_birthyear looks like this:
[1968, 1969, 1981, 1966, 1975, 1954, 1967, 1965, 1983, 1972]
Birth years of 2019’s highest paid actors
List Comprehension in Python
The previous example showed how to create a list from another list using a for loop. However, there is another approach we can use called “list comprehension”. It is an elegant way to create lists while avoiding the for-loop construct. With list comprehension, a new list can be created with a single line of code; this makes Python code easier to read and maintain.
So, rather than creating an empty list and adding each element to the end, we could initialize the list and its content at the same time, in the same statement, by using list comprehension.
In Python, syntax for list comprehension looks like this:
[ expression for item in a list {if condition_is_met} ]
List comprehension is defined inside square brackets [ ]. This reminds us that the resulting object is definitely a list.
Inside the brackets, an expression is defined. This is a statement that returns some specific value for each item in an existing list. With the code “for item in a list”, we are defining the sequence we want to iterate through. We can also define an if conditional statement inside the brackets to filter elements from an existing list.
Now that we know what list comprehension is, we can easily rewrite the for loop from our last example ...
import datetime highest_paid_actors_birthyear=[] for birthdate in highest_paid_actors_birthdate: birthdate_year = datetime.datetime.strptime(birthdate,"%d.%m.%Y").year highest_paid_actors_birthyear.append(birthdate_year)
… into something like this:
import datetime highest_paid_actors_birthyear = [datetime.datetime.strptime(birthdate,”%d.%m.%Y”).year for birthdate in highest_paid_actors_birthdate]
As you may have noticed, several lines of code in the for loop are not present in the list comprehension. For starters, with list comprehension we don't need to initialize an empty list, i.e. we don't need the statement highest_paid_actors_birthyear=[].
Using append() to tell Python that each new element should be added to the end is also unnecessary; we don't need to use append() in list comprehension. Instead, we are just defining the variable highest_paid_actors_birthyear and assigning the list comprehension result to that variable.
In our example, the expression is defined as:
datetime.datetime.strptime(birthdate,”%d.%m.%Y”).year
This is applied on each item in the list highest_paid_actors_birthdate. This statement returns the year for each birthdate and stores the results in a new list named highest_paid_actors_birthyear.
Code written like this is easier to understand. That’s why list comprehension is very famous and very popular with Python programmers.
Remember, we can also use a conditional if statement with list comprehension. We use if to select only certain elements that meet defined criteria. Here is one example:
[birthyear for birthyear in highest_paid_actors_birthyear if birthyear>1970]
Here we have the if statement if birthyear>1970. This means that Python will take only those elements (birthdate years) from the list highest_paid_actors_birthyear that are greater than 1970. Only on these elements will the expression be run, which means Python will return a filtered list.
The result will be a list containing four elements representing the birth years of the highest-paid actors of 2019 – but only when those actors were born after 1970:
[1981, 1975, 1983, 1972]
List comprehension with an if conditional statement
List Comprehension as an Alternative to Map()
List comprehension can replace the map() statement just as it does the for loop.
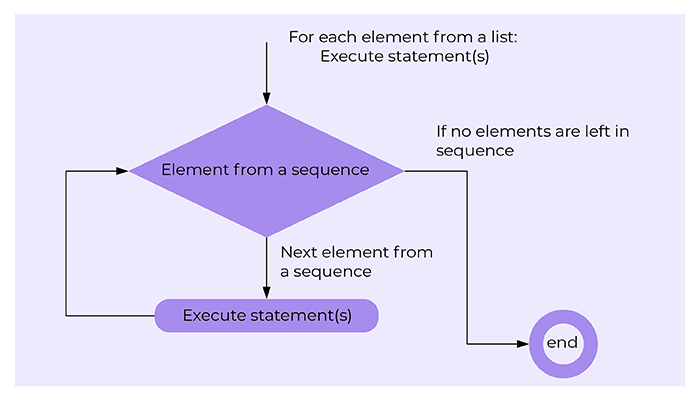
The map() statement applies a function to each element of a sequence
Map() is used when we want one function to apply to each element in a list. For example:
def calc_age(x): return datetime.date.today().year — x
actors_ages = map(age, highest_paid_actors_birthyear) list(actors_ages)
At the beginning of this code, we define the function calc_age(). By calling this function, Python will take a birth date year and return a person’s age. This is calculated as the current year minus the birth year. Now that we have the calc_age() function, we can use map() to apply that function to each element of highest_paid_actors_birthyear:
map(calc_age,highest_paid_actors_birthyear)
Map() returns a map object (actors_ages). If we want to see the contents (and we do), we need to apply the list() function (list(actors_ages)).
Now let’s see how this looks when we use list comprehension instead of a map() statement:
def calc_age(x): return datetime.date.today().year — x
actors_ages = [calc_age(birthyear) for birthyear in highest_paid_actors_birthyear]
Inside square brackets [ ], our expression is defined as calc_age(birthyear). The for birthyear in highest_paid_actors_birthyear part tells us the sequence through which we want to iterate.
Notice that we got the same result as using the map() function and we only needed one line of code outside of the function definition.
What Did We Learn About Python Lists and List Comprehension?
- A list is a sequence of one or more elements. In Python, it is created by placing all the elements inside square brackets. List items are separated by commas.
- Lists are changeable objects. After list creation, we can add, remove or change element(s).
- Lists are ordered and indexed, which means that each element is assigned a number indicating its position in the sequence. The element that is indexed with 0 is the first element in a list, the element with index 1 is the second, and so on.
- When we want to iterate through a whole list and apply code statement(s) to each element, we may use for loops or the map() function.
- List comprehension is an elegant alternative to for loops and the map() function. It allows us to iterate through a list and create a new list from an existing list with very little code.
In this article, we learned about list syntax, list comprehension syntax, and why it’s good to use list comprehension instead of map() or for loops. If you want to learn more about Python lists, I would suggest that you take the Vertabelo Academy Python courses mentioned above. Enjoy your learning and good luck working with lists and list comprehension!