Perfect! We can also create a tuple which describes the state of traffic lights:
# (red, yellow, green), True = light on
initial_state = (True, False, False)
The example above represents a red traffic light. Now, let's say the traffic lights follow the cycle shown below:
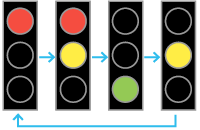
We can write a function which, given a tuple with the traffic lights' current state, returns the next state:
def next_lights_state(current_state):
red, yellow, green = current_state
if red:
if yellow:
return (False, False, True)
else:
return (True, True, False)
else:
if yellow:
return (True, False, False)
else:
return (False, True, False)
The function above checks which lights are on at the moment and returns the next traffic light setting.