Good job! In the previous part, we explained how you can compare two lists "element by element". In Python, there is another way to do this: the zip()
function. Take a look:
cars_dealer_a = [13000, 12300, 18900]
cars_dealer_b = [14000, 12000, 19000]
for car in zip(cars_dealer_a, cars_dealer_b):
print(car)
The code above produces the following output:
(13000, 14000)
(12300, 12000)
(18900, 19000)
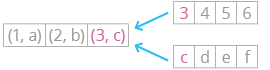
Here, we have two price lists of the same size: cars_dealer_a
and cars_dealer_b
. We want to compare the prices of specific cars for both dealers. To that end, we use the zip()
function. This function takes the first elements from both lists and makes them into a two-element tuple. Then, it takes the second elements from both lists and makes another two-element tuple, etc.
We can also write some more interesting code:
cars_dealer_a = [13000, 12300, 18900]
cars_dealer_b = [14000, 12000, 19000]
for car in zip(cars_dealer_a, cars_dealer_b):
if car[0] < car[1]:
print('Dealer A is cheaper by', car[1] - car[0])
elif car[1] < car[0]:
print('Dealer B is cheaper by', car[0] - car[1])
else:
print('Equal offers')
In the code above, we use the zip()
function to compare car prices for both dealers. For each pair of prices, we print which dealer offers a better price. Note that we used square brackets (car[0]
and car[1]
) to access the values from both lists.