Good job! Now let's see an example of counting with tuples as dictionary keys.
Suppose we have a rectangular board with various flowers growing in various cells:
flowers = [
((0, 3), 'violet'), ((1, 2), 'sunflower'), ((0, 3), 'forget-me-not'),
((3, 4), 'violet'), ((0, 3), 'poppy'), ((1, 2), 'forget-me-not')
]
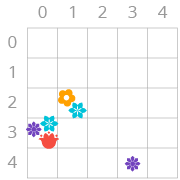
As you can see, there may be more than one flower in a given cell. We want to know how many flowers there are in each non-empty cell. Here's the code that will find the answer:
flower_counts = {}
for flower in flowers:
position, _ = flower
flower_counts[position] = flower_counts.get(position, 0) + 1
print(flower_counts)
Result:
{(0, 3): 3, (1, 2): 2, (3, 4): 1}
There are three flowers in the cell defined as (0, 3)
, two flowers in the cell (1, 2)
, and a single flower in (3, 4)
. Note that the keys in this example are tuples representing cell locations, but otherwise the entire code is similar to the previous examples.