Good job! Nested lists are also sometimes used to represent game boards. In the example below, we'll have a 4 x 5 board with either empty fields or fields with obstacles. The following board...
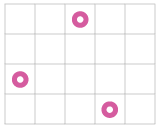
... can be represented in Python in the following way:
game_board = [
['-', '-', 'x', '-', '-'],
['-', '-', '-', '-', '-'],
['x', '-', '-', '-', '-'],
['-', '-', '-', 'x', '-']
]
A hyphen '-'
represents an empty field and an 'x'
represents a field with an obstacle.
Now, let's say we want to remove all the obstacles from the board. We can use the following code:
for i in range(len(game_board)):
for j in range(len(game_board[i])):
if game_board[i][j] == 'x':
game_board[i][j] = '-'
The code above checks every field in the board. If the field contains an obstacle (an 'x'
), it turns it into an empty field ('-'
).